Common Errors
Authentication and Authorization Issues (401)
These errors are commonly referred to as 401
errors in the system and can be due to the following:
- Missing or Invalid Tokens: Ensure that valid API keys or tokens are included in each request.
- Expired access tokens: Check if your token has expired and refresh when necessary.
- Revoked access tokens: Admins can revoke access tokens for security and abuse-related cases. Users can also revoke access tokens on the eezi application.
- Incorrect Scopes or Permissions: eezi utilizes fine-grained scope access control to prevent unauthorized access or modification of data.
{
"message":"Unauthorized"
}
{
"error": {
"message": "Unauthorized",
"description": "Missing the following required scope to access this resource: invoice:read",
"status": 401
}
}
Rate Limit Exceeded (429 Too Many Requests)
Rate limits act as an additional level of protection for downstream partners and receiving parties.
{
"message":"Too Many Requests"
}
Invalid parameters
Incorrect data will cause schema validation, logic validation, or downstream routing errors. As per the example below, errors will be indicated by an error key.
{
"ref":"DCINV0XB8KWMPQVA68YT",
// ...invoice fields without a total
"error":{
"name":"VALIDATION_SCHEMA",
"status":400,
"message":"Invalid invoice",
"description":"\"total\" is required"
}
}
{
"ref":"DCINVITA0YSKJ22D5TSL",
// ...invoice fields with invalid total
"error":{
"name":"VALIDATION_LOGIC",
"status":400,
"message":"Invalid invoice",
"description":"Invoice total does not match line items."
}
}
{
"ref":"E2E-7c594bf4-e512-4dea-936d-e8c2b93947df",
// ...invoice fields
"error":{
"name":"TAX_AUTHORITY_INVOICE_CREATE",
"status":400,
"message":"Invoice rejected",
"description":"The document date is less than the last generated document date for the series name. Document date specified 2024-03-29 00:00:00, last document date: 2024-03-31 00:00:00, series name: SPINV15."
}
}
Timeouts and Slow Responses
Various systems are involved to and from the receiving party when submitting invoices and other documents. In some cases, parallel routing and distribution post submission for mandated regions. To timeouts and slow responses, we implemented the following mitigating steps:
- Artificial and enforced timeouts: eezi automatically enforces timeouts after 29 seconds and responds with a timeout error
- 202 Accepted responses: For known long-running tasks or notoriously slow downstream systems, eezi will return 202 responses after applying its best effort to try and finalize a response.
- Read endpoints: The invoice and credit not read endpoints can be used to get the latest state of the document. We recommend calling the read endpoint in 30-second intervals to prevent overwhelming downstream systems and partners.
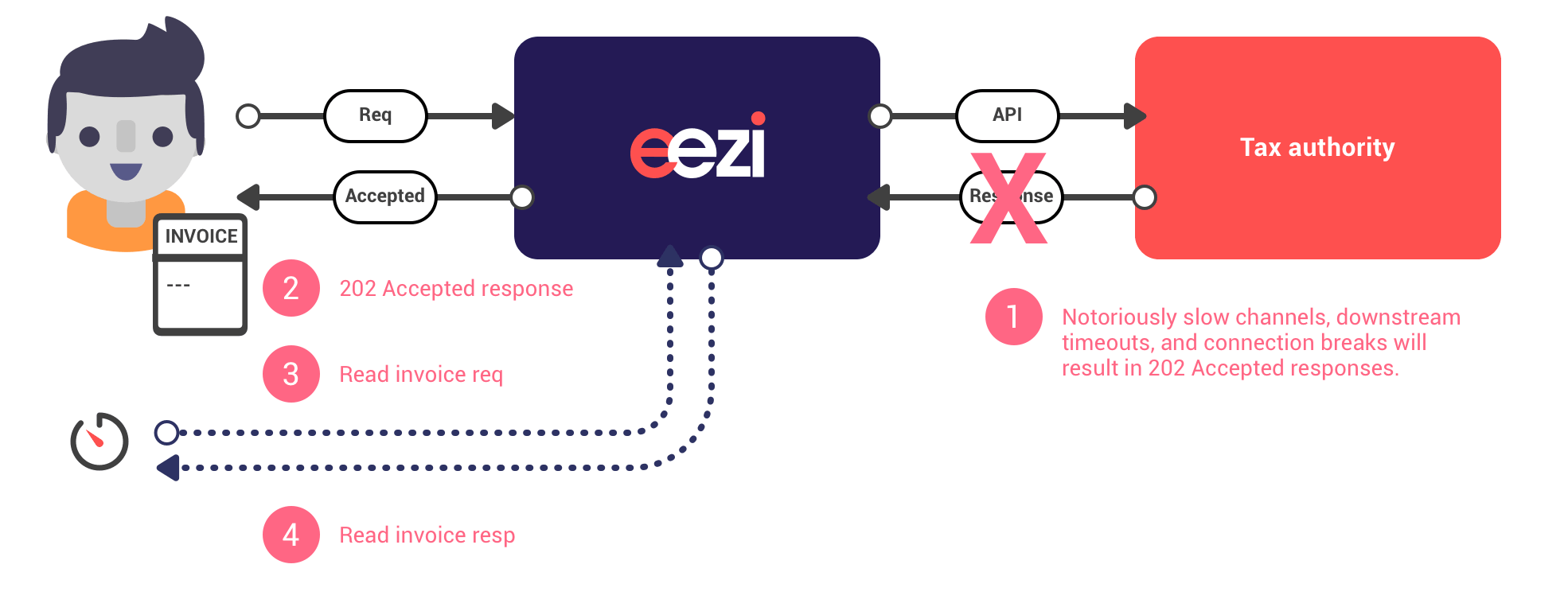
Test environments and simulated error responses
All eezi mock and test environments feature simulated error responses to several distinct error types for reliable testing of both positive and negative scenarios. Some are triggered by invalid data (schema or logic), and others can be forced by setting the total
amount to specific values.
Success and Accepted - 2xx responses
Both VALIDATION_SCHEMA
and VALIDATION_LOGIC
share the same HTTP status of 400.
Status | Description | How to Trigger |
---|---|---|
201 | Successfully created | Send a total value outside the 1202.xx, 403.xx, 429.xx rangesAlternatively, you can explicitly trigger this by sending a total value of 201 |
200 | Action was successful | Applies to actions like read after a create |
202 | Request was accepted, but still pending a response. (This simulates long running tasks, real or enforced timeouts) This will resolve to a success. | Send a total value of 202.00 , wait more than 10 seconds and read invoice or credit note. |
1202 | Request was accepted, but still pending a response. (This simulates long running tasks, real or enforced timeouts) This will resolve to an error. | Send a total value of 1202.00 , wait more than 10 seconds and read invoice or credit note. |
Schema or Logic validation - 400 Errors
Both VALIDATION_SCHEMA
and VALIDATION_LOGIC
share the same HTTP status of 400.
Error Key | Message | Description | How to Trigger |
---|---|---|---|
VALIDATION_SCHEMA | Bad Request | Request does not match schema | Omit required fields (e.g., total , line item details).Provide invalid data format. Send structurally invalid JSON. |
VALIDATION_LOGIC | Bad Request | Invalid request logic | Mismatch between line-item sums and total .Negative prices or inconsistent currency totals. Other logical discrepancies. |
Miscellaneous - 403 Errors
Several different error scenarios fall under HTTP 403. Some can be forced in Test by setting the total
to specific values (403.xx
, 403.01
).
Error Key | Message | Description | How to Trigger |
---|---|---|---|
DUPLICATE_REF | Duplicate Ref | A document with the same ref already exists. | Attempt to create an invoice or credit note with a ref that is already in use. |
COUNTRY_CONFIGURATION | Country not configured | Current organisation country configuration is incomplete. | By default, total = 403.xx forces this error in Test.Example: total = 403.20 . |
FRAUD_DETECTED | Potential fraud detected | This transaction was flagged for potential fraud. | Specifically triggered by total = 403.01 . |
Not found - 404 Errors
Error Key | Message | Description | How to Trigger |
---|---|---|---|
NOT_FOUND | Not found | Item not found | Attempt to read or fetch an invoice/credit note using an invalid or non-existent id or ref .Example: GET /invoices/invalid-id . |
Rate limiting or Throttling - 429 Errors
Error Key | Message | Description | How to Trigger |
---|---|---|---|
TOO_MANY_REQUESTS | Too Many Requests | Request limit exceeded | For testing, set total = 429.xx (e.g., total = 429.10 ). |
Downstream - 503 Errors
These errors indicate downstream or external integration issues (e.g., with tax authorities). You can simulate many of these by setting total
to specific values (503.xx
, 1202.xx
) or by meeting timing conditions (request older than 10 seconds).
Error Key | Message | Description | How to Trigger |
---|---|---|---|
TAX_AUTHORITY_INVOICE_CREATE | Downstream error | The invoice could not be sent to the recipient. | total = 503.xx : Forces a generic “Error processing document” scenario.total = 503.01 : “Tax ID does not match company legal name.”total = 503.03 : “The invoice could not be sent to the recipient.”total = 1202.xx and request > 10 seconds old: “Error processing document” scenario. |
TAX_AUTHORITY_CREDIT_NOTE_CREATE | Downstream error | The credit note could not be sent to the recipient. | Same triggers as TAX_AUTHORITY_INVOICE_CREATE , but applies to credit notes. |
TAX_AUTHORITY_UNAUTHORISED | Downstream error | Customer integration details are invalid. | Triggered by total = 503.02 . |
Quick Reference Table: Forced Errors by total
Value
total
ValueUse the table below to see which errors can be intentionally forced in the Test environment by setting the total
amount.
Forced total | Status | Message |
---|---|---|
201 | 201 | Successfully created |
202.xx (resolves 10s later) | 202 (Resolves to 200) | 202 Accepted that results in a success when read more than 10 seconds later. |
1202.xx (resolves 10s later) | 202 (Resolves to 503) | 202 Accepted that results in an error when read more than 10 seconds later. |
403.xx (e.g., 403.20 ) | 403 | Country not configured |
403.01 | 403 | Potential fraud detected |
429.xx (e.g., 429.10 ) | 429 | Too Many Requests |
503.xx (e.g., 503.15 ) | 503 | Error processing document |
503.01 | 503 | Tax ID does not match company legal name |
503.02 | 503 | Customer integration details are invalid |
503.03 | 503 | Could not be sent to the recipient |
Notes:
- For the
202.xx
and1202.xx
triggers, you must also ensure the request is over 10 seconds old to see the final status and message when fetching the document.
Updated 4 months ago